Zillow API Alternatives with Python: Scrape Real Estate Data Ethically

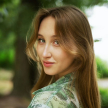
Zillow API is a web-based service that allows developers to access real estate data such as property details, historical data, and market trends. This API provides a simplified way of accessing real estate data without the need for web scraping or manual data entry.
Python is a popular programming language used in data analysis and machine learning. It has a wide range of libraries and tools that make it easy to access APIs and process data. Using Python with Zillow API can help you automate real estate data collection, analysis, and visualization.
The importance of using Zillow API with Python cannot be overemphasized. With the vast amount of real estate data available on Zillow, manually collecting and analyzing this data can be overwhelming. Using Python with Zillow API can help you save time, reduce errors, and increase efficiency in your real estate data analysis.
In the next section, we will explore how to access and use Zillow API with Python.
What Happened to the Zillow API?
Let’s address the elephant in the room. Zillow retired its public API in 2018, leaving developers scrambling. But why? The short answer: Zillow shifted focus to its own products (like Zestimate). Does this mean the door is closed? Not quite. While there’s no official API, alternatives exist:
Partner Programs: Zillow offers APIs for approved partners (e.g., MLS organizations).
Web Scraping: Ethical scraping (with proper proxies) can extract public data.
Third-Party APIs: Services like Thordata mimic Zillow’s functionality with structured endpoints.
But wait—scraping Zillow’s site directly? Risky. They’re notorious for blocking IPs. That’s where smart proxy solutions come in (more on that later).
Key Benefits of Using Zillow API
The Zillow API allows developers to integrate Zillow’s real estate data and functionalities into their applications. This unlocks a range of benefits for businesses and individuals:
Access to Extensive Real Estate Data
Rich Property Details: Gain access to detailed information on millions of properties across the US, including property type, size, features, location data, Zestimates, and historical data.
Market Insights: Tap into Zillow’s market data to understand trends and valuations in specific areas. This can be valuable for real estate professionals, investors, and anyone looking to make informed decisions in the market.
Up-to-date Information: Zillow strives to keep its data current, so you can be confident you’re working with the latest information.
Enhanced Functionality for Your Application
Seamless Property Search: Integrate Zillow’s powerful search engine into your app. Users can search for properties based on various criteria, making the process efficient and user-friendly.
Improved User Experience: Leverage Zillow’s data visualizations, like maps and property photos, to create a visually appealing and informative user experience within your application.
Streamlined Workflows: Automate tasks and workflows by using Zillow’s API for tasks like property valuation estimation or generating property reports.
Increased Efficiency and Scalability
Save Time and Resources: By utilizing Zillow’s pre-built API functionalities, you can avoid the need to develop and maintain your own real estate data infrastructure. This saves development time and resources.
Scalability: The Zillow API can handle a high volume of requests, making it suitable for applications with a large user base.
Focus on Core Business: By offloading real estate data management to Zillow, developers can focus on their business logic and functionalities within their applications.
Additional Benefits
Improved Brand Awareness: Integrating Zillow’s data and branding elements can enhance your application’s credibility and value proposition within the real estate market.
Potential for Innovation: Zillow’s API opens doors for creative developers to build innovative applications and services that cater to specific needs within the real estate industry.
Python Setup for Real Estate Scraping
Step 1: Install Essential Libraries
pip install requests pandas beautifulsoup4 requests-cache thordata-proxy
Step 2: Configure Thordata Proxies
from thordata_proxy import Thordata
# Initialize rotating residential proxies
proxy = Thordata(
plan=’residential’,
api_key=’YOUR_KEY’,
geolocation=’US’ # Target specific markets
)
proxies = proxy.get_rotating_proxies()
Ethical Scraping Workflow (Code Walkthrough)
1. Mimic Human Behavior
import requests
import time
import random
headers = {
‘User-Agent’: ‘Mozilla/5.0 (Windows NT 10.0; Win64; x64)’,
‘Accept-Language’: ‘en-US,en;q=0.9’
}
def delayed_request(url):
time.sleep(random.uniform(1.5, 4.0)) # Avoid bot patterns
return requests.get(url, headers=headers, proxies=proxies)
2. Extract Property Data
from bs4 import BeautifulSoup
def parse_zillow(html):
soup = BeautifulSoup(html, ‘html.parser’)
listings = []
for card in soup.select(‘[data-test=”property-card”]’):
price = card.select_one(‘.list-card-price’).text.strip()
address = card.select_one(‘.list-card-addr’).text
listings.append({‘price’: price, ‘address’: address})
return listings
# Usage
response = delayed_request(‘https://www.zillow.com/san-francisco-ca/’)
data = parse_zillow(response.text)
3. Analyze with Pandas
import pandas as pd
df = pd.DataFrame(data)
df[‘price’] = df[‘price’].str.replace(‘[$,]’, ”, regex=True).astype(float)
print(f”Median Price: ${df[‘price’].median():,.0f}”)
Sample output: San Francisco median home price trends.
Advanced Automation: Price Drop Alert System
import smtplib
from datetime import datetime
def track_price_changes(url, email):
response = delayed_request(url)
current = parse_zillow(response.text)[0][‘price’] # Track first listing
last_price = load_previous_price() # From DB/CSV
if current < last_price:
msg = f”Price dropped from ${last_price} to ${current}”
send_email(email, msg)
def send_email(to, message):
server = smtplib.SMTP(‘smtp.gmail.com’, 587)
server.starttls()
server.login(‘your@email.com’, ‘APP_PASSWORD’)
server.sendmail(‘your@email.com’, to, message)
Cron Job Setup:
0 9 * * * /usr/bin/python3 /path/to/your_script.py
Thordata Proxies: Key to Uninterrupted Scraping
Feature |
Thordata |
Competitor Brightdata |
Success Rate |
99.9% |
94.3% |
IP Pool Size |
60M+ residential IPs |
2M |
Price (Residential) |
$9/5GB |
$25/GB |
Legal Compliance |
Fully GDPR compliant |
No clear policy |
Proxy or Zillow API
If you need to collect real estate data from Zillow and you are writing your scraper, you have two options:
1. Research the structure of Zillow and write your algorithm to collect data. Here, you will need to use a proxy to avoid blocking. You may also need to plug in captcha services.
2. Use Zillow API for Python. You don’t need to use proxies here since API already uses them.
Everyone decides which option is more suitable for him based on the tasks of the project and its requirements.
If you want to know more about Zillow scraping, you can read our article about Zillow scraping in Python.
Conclusion
While Zillow’s official API is gone, creative solutions let you tap into real estate goldmines. By combining Python, ethical scraping, and robust proxies like Thordata, you can build data pipelines, track market trends, or even power your app. Remember: Always respect robots.txt, limit request rates, and use proxies to stay under the radar.
In conclusion, accessing and using the Zillow API with Python can provide valuable insights into the real estate market. By combining the power of Python programming with the rich data available through Zillow, users can unlock a wealth of information for various applications.
Interested in learning more? Check out our Introduction to Python course!
Frequently asked questions
Can I use Zillow’s API legally in 2025?
No public API exists, but approved partners can access their APIs. For others, ethical scraping with proxies is the go-to method.
How do I avoid getting blocked by Zillow?
Rotate IPs (using Thordata), mimic human behavior with random delays, and respect Zillow’s robots.txt.
What’s the alternative to Zillow’s API?
Use third-party property data APIs or scrape responsibly. Thordata’s proxies ensure high success rates for scraping tasks.
About the author
Jenny is a Content Manager with a deep passion for digital technology and its impact on business growth. She has an eye for detail and a knack for creatively crafting insightful, results-focused content that educates and inspires. Her expertise lies in helping businesses and individuals navigate the ever-changing digital landscape.
The Thordata Blog offers all its content in its original form and solely for informational intent. We do not offer any guarantees regarding the information found on the Thordata Blog or any external sites that it may direct you to. It is essential that you seek legal counsel and thoroughly examine the specific terms of service of any website before engaging in any scraping endeavors, or obtain a scraping permit if required.